In the Django framework:
- A project is a Django installation with some settings.
- An application is a group of models, views, templates, and URLs.
Django 프로젝트에는 하나 이상의 응용 프로그램이 있을 수 있습니다. 예를 들어 프로젝트는 블로그, 사용자 및 위키와 같은 여러 응용 프로그램으로 구성될 수 있는 웹 사이트와 같습니다.
일반적으로 다른 Django 프로젝트에서 재사용할 수 있는 Django 애플리케이션을 디자인합니다. 다음 그림은 Django 프로젝트의 구조와 응용 프로그램을 보여줍니다.
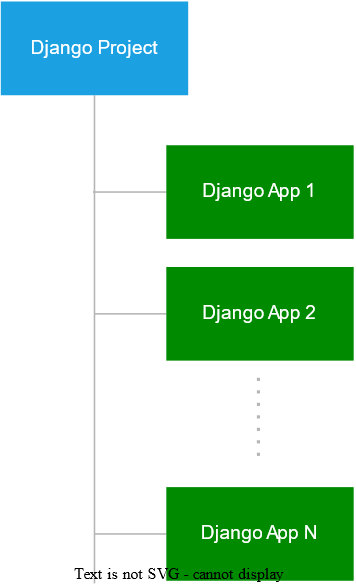
Creating a blog application
명령어 : python manage.py startapp app_name
이 명령은 일부 파일이 있는 블로그 디렉터리를 만듭니다.
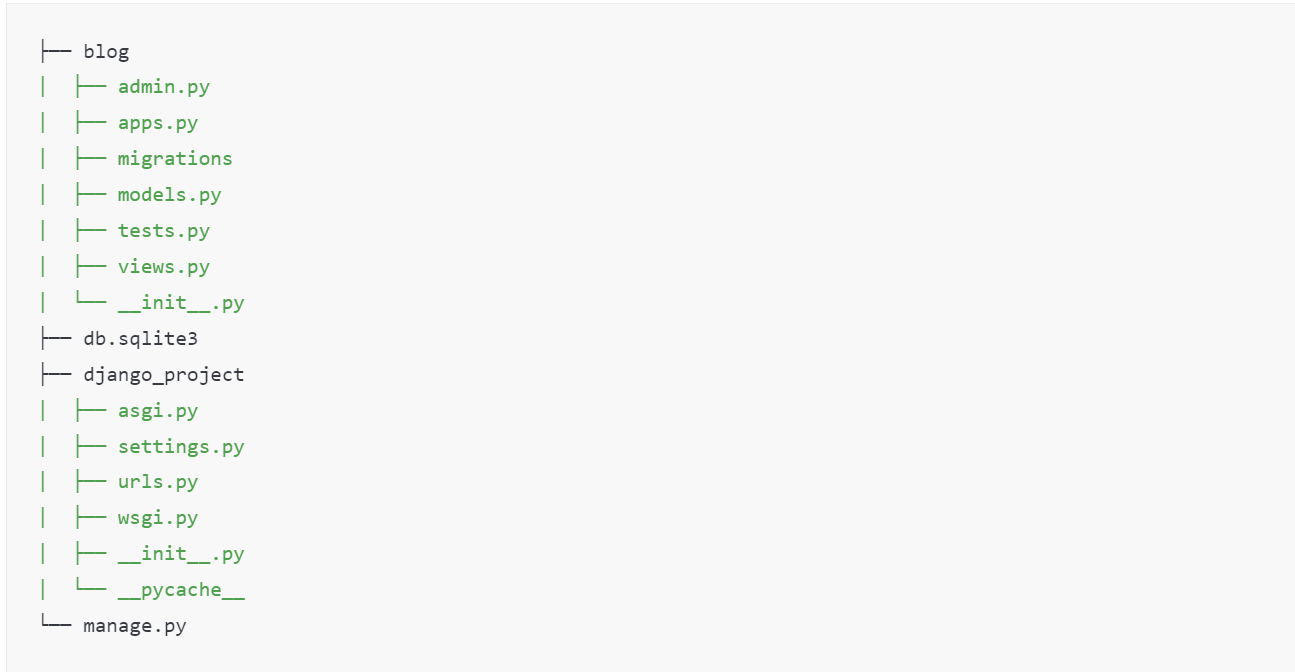
Registering an application
응용 프로그램을 만든 후에는 특히 응용 프로그램에서 템플릿을 사용하고 데이터베이스와 상호 작용하는 경우 프로젝트에 등록해야 합니다.
blog > apps.py
from django.apps import AppConfig
class BlogConfig(AppConfig):
default_auto_field = 'django.db.models.BigAutoField'
name = 'blog'
App등록 : INSTALLED_APPS 목록에 blog.apps.BlogConfig 클래스를 추가합니다 .
django_project > settings.py
INSTALLED_APPS = [
# ...
'blog.apps.BlogConfig',
]
또는 다음과 같이 INSTALLED_APPS 목록에서 blog와 같은 앱 이름을 사용할 수 있습니다.
INSTALLED_APPS = [
# ...
'blog',
]
Creating a view
blog 디렉터리의 views.py 파일에는 다음 기본 코드가 함께 제공됩니다.
blog > views.py
from django.shortcuts import render
# Create your views here.
A view is a function that takes an HttpRequest
object and returns an HttpResponse
object.
To create a new view, you import the HttpResponse
from the django.http
into the views.py
file and define a new function that accepts an instance of the HttpRequest
class:
from django.shortcuts import render
from django.http import HttpResponse
def home(request):
return HttpResponse('<h1>Blog Home</h1>')
이 예제에서 home() 함수는 HTML 코드가 포함된 새 HttpResponse 객체를 반환합니다. HTML 코드에는 h1 태그가 포함되어 있습니다.
home() 함수는 HttpRequest 객체 의 인스턴스를 받아들이고 HttpResponse 객체를 반환합니다. 이를 함수 기반 뷰라고 합니다. 나중에 클래스 기반 view를 만드는 방법을 배웁니다.
home() 함수를 사용하여 URL을 매핑하려면 블로그 디렉터리 내에 새 파일 urls.py 만들고 urls.py 파일에 다음 코드를 추가합니다 .
blog > urls.py
from django.urls import path
from . import views
urlpatterns = [
path('', views.home, name='posts'),
]
path() 함수를 사용하여 블로그 URL을 home() 함수와 매핑하는 경로를 정의합니다 .
name 키워드 인수는 경로의 이름을 정의합니다. 나중에 blog/와 같은 하드 코드 URL을 사용하는 대신 게시물 이름을 사용하여 URL을 참조할 수 있습니다.
경로 이름을 사용하면 모든 곳에서 하드 코딩된 URL을 변경하는 대신 경로의 URL을 urls.py 에서 my-blog/와 같은 다른 것으로 변경할 수 있습니다.
경로의 마지막 인수는 name='posts'와 같은 키워드 인수여야 합니다.
다음과 같이 위치 인수를 사용하는 경우 에러 발생 :
from django.urls import path
from . import views
urlpatterns = [
path('', views.home, 'posts'), # Error
]
블로그의 경로가 작동하도록하려면 Django 프로젝트의 urls.py 파일에 블로그 응용 프로그램의 urls.py 포함시켜야합니다.
django_project > urls.py
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('blog/', include('blog.urls')),
]
프로젝트 urls.py 에서는 django.urls에서 include 함수를 import하고 블로그 경로를 blog.urls에 매핑합니다.
이렇게하면 http://127.0.0.1:8000/blog/ 로 이동할 때 Django는 views.py 모듈의 home() 함수를 실행하고 h1 태그를 표시하는 웹 페이지를 반환합니다.
http://127.0.0.1:8000/blog/ 로 이동하면 Blog Home heading 이 표시된 웹 페이지가 표시됩니다.
동작방식:
- First, the web browser sends an HTTP request to the URL http://127.0.0.1:8000/blog/
- Second, Django executes the urls.py in the django_project directory. It matches the blog/ with the URL in the urlpatterns list in the urls.py. As a result, it sends '' to the urls.py of the blog app.
- Third, Django runs the urls.py file in the blog application. It matches the '' URL with the views.home function and execute it, which returns an HTTP response that outputs a h1 tag.
- Finally, Django returns a webpage to the web browser.
Adding more routes
First, define the about()
function in the views.py
of the blog
application:
from django.shortcuts import render
from django.http import HttpResponse
def home(request):
return HttpResponse('<h1>Blog Home</h1>')
def about(request):
return HttpResponse('<h1>About</h1>')
Second, add a route to the urls.py
file:
from django.urls import path
from . import views
urlpatterns = [
path('', views.home, name='posts'),
path('about/', views.about, name='about'),
]
Third, open the URL http://127.0.0.1:8000/blog/about/
, and you’ll see a page that displays the about page.
Now, if you open the home URL, you’ll see a page that displays a page not found with a 404 HTTP status code.
The reason is that the urls.py
in the django_project
doesn’t have any route that maps the home URL with a view.
To make the blog application the homepage, you can change the route from blog/
to ''
as follows:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('blog.urls')),
]
If you open the URL http://127.0.0.1:8000
, you’ll see the blog home page. And navigating to the URL http://127.0.0.1:8000/about/
will take you to the about page.
Download the Django Project source code
Summary
- A Django project contains one or more applications.
- A Django application is a group of models, views, templates, and URLs.
- Use
python manage.py startapp app_name
command to create a new Django application. - Define a function in the
views.py
file to create a function-based view. - Define a route in the
urls.py
file of the application to map a URL pattern with a view function. - Use the
include()
function to include theurls.py
of app in theurls.py
of the project.
'Django11' 카테고리의 다른 글
Django Migrations (0) | 2023.04.28 |
---|---|
Django Models (0) | 2023.04.28 |
Django 배포 (0) | 2023.04.14 |
Getting Started with Django (0) | 2023.04.08 |
[ DJANGO ] Index (0) | 2023.04.08 |
댓글