출처 :https://www.pythontutorial.net/python-oop/python-single-responsibility-principle/
Python Single Responsibility Principle
In this tutorial, you'll learn about the Single Responsibility Principle and how to implement it in Python.
www.pythontutorial.net
What is SOLID
SOLID는 Uncle Bob에 의한 5 가지 소프트웨어 디자인 원칙을 나타내는 약어입니다.
- S – Single responsibility Principle
- O – Open-closed Principle
- L – Liskov Substitution Principle
- I – Interface Segregation Principle
- D – Dependency Inversion Principle
Introduction to the single responsibility principle
SRP(단일 책임 원칙)에 따르면 모든 클래스, 메서드 및 함수는 하나의 작업 또는 변경할 이유가 하나만 있어야 합니다.
단일 책임 원칙의 목적은 다음과 같습니다.
- 응집력이 높고 강력한 클래스, 메서드 및 함수를 만듭니다.
- class composition촉진
- 코드 중복 방지
다음 Person 클래스를 살펴보겠습니다.
class Person:
def __init__(self, name):
self.name = name
def __repr__(self):
return f'Person(name={self.name})'
@classmethod
def save(cls, person):
print(f'Save the {person} to the database')
if __name__ == '__main__':
p = Person('John Doe')
Person.save(p)
이 Person 클래스에는 두 가지 작업이 있습니다.
- 사용자의 property를 관리합니다.
- 데이터베이스에 사람을 저장합니다.
나중에 Person을 파일과 같은 다른 저장소에 저장하려면 save() 메서드를 변경해야 하며 이 경우 전체 Person 클래스도 변경됩니다.
Person 클래스가 단일 책임 원칙을 준수하도록 하려면 Person을 데이터베이스에 저장하는 다른 클래스를 만들어야 합니다. 예를 들어:
class Person:
def __init__(self, name):
self.name = name
def __repr__(self):
return f'Person(name={self.name})'
class PersonDB:
def save(self, person):
print(f'Save the {person} to the database')
if __name__ == '__main__':
p = Person('John Doe')
db = PersonDB()
db.save(p)
이 디자인에서는 Person 클래스를 Person 및 PersonDB의 두 클래스로 구분합니다.
- Person 클래스는 사용자의 속성을 관리합니다.
- PersonDB 클래스는 데이터베이스에 Person을 저장하는 역할을 합니다.
이 디자인에서는 Person을 다른 저장소에 저장하려는 경우 이를 수행할 다른 클래스를 정의할 수 있습니다. 그리고 Person 클래스를 변경할 필요가 없습니다.
클래스를 디자인할 때는 변경 이유가 같은 관련 메서드를 함께 배치해야 합니다. 즉, 클래스가 다른 이유로 변경되는 경우 클래스를 분리해야합니다.
이 디자인에는 Person 및 PersonDB의 두 클래스를 처리해야 하는 한 가지 문제가 있습니다.
보다 편리하게 만들려면 다음과 같이 Person 클래스가 PersonDB 클래스의 외관이 되도록 facade pattern(외관 패턴)을 사용할 수 있습니다.
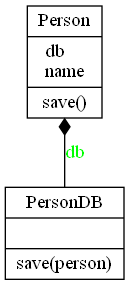
class PersonDB:
def save(self, person):
print(f'Save the {person} to the database')
class Person:
def __init__(self, name):
self.name = name
self.db = PersonDB()
def __repr__(self):
return f'Person(name={self.name})'
def save(self):
self.db.save(person=self)
if __name__ == '__main__':
p = Person('John Doe')
p.save()
Summary
- SRP(단일 책임 원칙) - 모든 클래스, 메서드 또는 함수가 하나의 작업 또는 변경할 이유가 하나만 있어야 한다.
- 단일 책임 원칙을 사용하여 클래스, 메서드 및 함수를 동일한 변경 이유로 구분합니다.
'Python Object-oriented Programming' 카테고리의 다른 글
Liskov Substitution Principle (0) | 2023.04.04 |
---|---|
Open-closed Principle (0) | 2023.04.04 |
enum-auto (0) | 2023.04.04 |
Customize and Extend Python Enum Class (0) | 2023.04.04 |
num aliases & @enum.unique Decorator (0) | 2023.04.04 |
댓글