Model-View-Controller framework for Smalltalk (Krasner and Pope, 1988), which divided the user interface problem into three parts. The parts were referred to as a data model, containing the computational parts of the program; the view, which presents the user interface; and the controller, which interacts between the user and the view
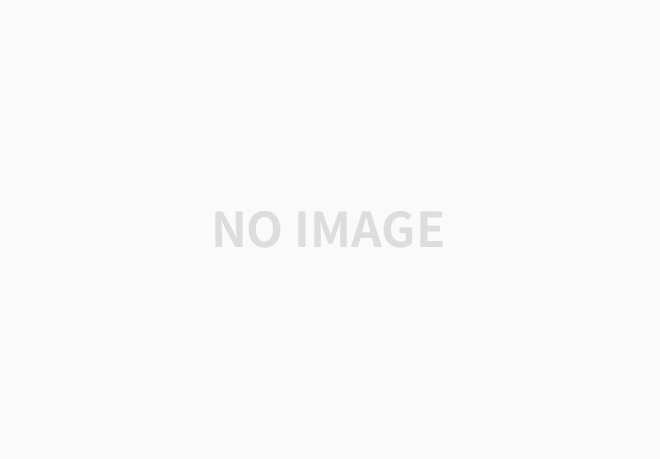
The authors divided these patterns into three types: creational, structural, and behavioral.
- Creational patterns create objects for you instead of having you instantiate objects directly. This gives your program more flexibility in deciding which objects need to be created for a given case.
- Structural patterns help you compose groups of objects into larger structures, such as complex user interfaces or accounting data.
- Behavioral patterns help you define the communication between objects in your system and control the flow in a complex program.
The Learning Process
We have found that learning design patterns is a multiple-step process:
- Acceptance
- Recognition
- Internalization
Notes on Object-Oriented Approaches
디자인 패턴을 사용하는 근본적인 이유는 클래스를 분리하여 서로에 대해 너무 많이 알 필요가 없도록 하기 위해서입니다. 마찬가지로 중요한 것은 이러한 패턴을 사용하면 클래스를 재창조하지 않고 다른 프로그래머가 쉽게 이해할 수있는 용어로 프로그래밍 접근 방식을 간결하게 설명 할 수 있다는 것입니다.
OO 프로그래머가 이러한 분리를 달성하기 위해 사용하는 여러 가지 전략이 있으며, 그 중 캡슐화 및 상속이 있습니다.
- Design Patterns suggests that you always Program to an interface and not to an implementation.
Putting this more succinctly, you should define the top of any class hierarchy with an abstract class or an interface, which implements no methods but simply defines the methods that class will support. Then in all your derived classes, you have more freedom to implement these methods as best suits your purposes.
Python does not directly support interfaces, but it does let you write abstract classes, where the methods have no implementation. Remember the comd interface to the DButton class:
class DButton(Button):
def __init__(self, master, **kwargs):
super().__init__(master, **kwargs)
super().config(command=self.comd)
# abstract method to be called by children
def comd(self): pass
This is a good example of an abstract class. Here you fill in the code for the command method in the derived button classes. As you will see, it is also an example of the Command design pattern.
- The other major concept you should recognize is object composition.
We have already seen this approach in the Statelist examples. Object composition is simply the construction of objects that contain others—the encapsulation of several objects inside another one. Many beginning OO programmers tend to use inheritance to solve every problem, but as you begin to write more elaborate programs, the merits of object composition become apparent. Your new object can have the interface that works best for what you want to accomplish without having all the methods of the parent classes.
Thus, the second major precept suggested by Design Patterns is Favor object composition over inheritance.
At first this seems contrary to the customs of OO programming, but you will see any number of cases among the design patterns where we find that inclusion of one or more objects inside another is the preferred method.
Python Design Patterns
The following chapters discuss each of the 23 design patterns featured in the Design Patterns book, along with at least one working program example for that pattern. The programs have some sort of visual interface as well to make them more immediate to you.
Which design patterns are most useful? This depends on the individual programmer. The ones we use the most are Command, Factory, Decorator, Facade, and Mediator, but we have used nearly every one at some point.
References
- Erich Gamma, Object-Oriented Software Development based on ET++, (in German) (Springer-Verlag, Berlin, 1992).
- Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides, Design Patterns, Elements of Reusable Object-Oriented Software (Reading, MA: Addison-Wesley, 1995).
Design Pattern 원조 Book (GOF - Gang of Four) : Design Patterns: Elements of Reusable Software - James Cooper, Java Design Patterns: A Tutorial (Boston: Addison-Wesley: 2000).
- James Cooper, C# Design Patterns: A Tutorial (Boston: Addison-Wesley, 2003).
- Brandon Rhodes, “Python Design Patterns”, https://python-patterns.guide.
'James Cooper - Python Programming with D' 카테고리의 다른 글
The Factory Method Pattern (0) | 2023.03.23 |
---|---|
The Factory Pattern (0) | 2023.03.23 |
Visual Programming of Tables of Data (0) | 2023.03.23 |
Visual Programming in Python (0) | 2023.03.23 |
Introduction (0) | 2023.03.23 |
댓글