출처 : https://www.pythontutorial.net/python-oop/python-multiple-inheritance/
Python Multiple Inheritance
In this tutorial, you'll learn about Python multiple inheritance and how method order resolution works in Python.
www.pythontutorial.net
Introduction to the Python Multiple inheritance.
클래스가 단일 클래스에서 상속되면 단일 상속이다. Python을 사용하면 클래스가 여러 클래스에서 상속받을 수 있습니다. 클래스가 둘 이상의 클래스에서 상속되는 경우 multiple inheritance을 갖게 됩니다.
여러 클래스를 확장하려면 다음과 같이 자식 클래스의 클래스 이름 뒤에 괄호 () 안에 부모 클래스를 지정합니다.
class ChildClass(ParentClass1, ParentClass2, ParentClass3):
pass
다중 상속에 대한 구문은 클래스 정의의 매개 변수 목록과 비슷합니다. 괄호 안에 하나의 부모 클래스를 포함하는 대신 쉼표로 구분된 두 개 이상의 클래스를 포함합니다.
다중 상속이 어떻게 작동하는지 이해하기 위해 예를 들어 보겠습니다.
먼저 go() 메서드가 있는 Car 클래스를 정의합니다.
class Car:
def go(self):
print('Going')>
둘째, fly() 메서드가 있는 Flyable 클래스를 정의합니다.
class Flyable:
def fly(self):
print('Flying')
셋째, Car 및 Flyable 클래스 모두에서 상속되는 FlyingCar를 정의합니다.
class FlyingCar(Flyable, Car):
pass
FlyingCar는 Car 및 Flyable 클래스에서 상속되므로 해당 클래스의 메서드를 재사용합니다. 즉, 다음과 같이 FlyingCar 클래스의 인스턴스에서 go() 및 fly() 메서드를 호출할 수 있습니다.
if __name__ == '__main__':
fc = FlyingCar()
fc.go()
fc.fly()
Output:
Going
Flying
Method resolution order (MRO)
부모 클래스에 동일한 이름의 메서드가 있고 자식 클래스가 메서드를 호출하는 경우 Python은 MRO(메서드 확인 순서)를 사용하여 호출할 올바른 메서드를 검색합니다. 다음 예제를 고려하십시오.
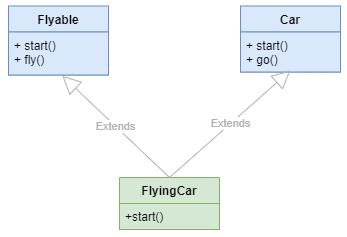
먼저 start() 메서드를 Car, Flyable 및 FlyingCar 클래스에 추가합니다 . FlyingCar 클래스의 start() 메서드에서 super()의 start() 메서드를 호출합니다.
class Car:
def start(self):
print('Start the Car')
def go(self):
print('Going')
class Flyable:
def start(self):
print('Start the Flyable object')
def fly(self):
print('Flying')
class FlyingCar(Flyable, Car):
def start(self):
super().start()
둘째, FlyingCar 클래스의 인스턴스를 만들고 start() 메서드를 호출합니다.
if __name__ == '__main__':
car = FlyingCar()
car.start()
Output:
Start the Flyable object
출력에서 명확하게 알 수 있듯이 super().start()는 Flyable 클래스의 start() 메서드를 호출 합니다.
다음은 FlyingCar 클래스의 __mro__ 보여줍니다.
print(FlyingCar.__mro__)
Output:
(<class '__main__.FlyingCar'>, <class '__main__.Flyable'>, <class '__main__.Car'>, <class 'object'>)
왼쪽에서 오른쪽으로 FlyingCar, Flyable, Car, object가 표시됩니다.
Car 및 Flyable 객체는 객체 클래스에서 암시적으로 상속됩니다. FlyingCar의 객체에서 start() 메서드를 호출하면 파이썬은 __mro__ 클래스 검색 경로를 사용합니다.
Flyable 클래스는 FlyingCar 클래스 다음이므로 super().start()는 FlyingCar 클래스의 start() 메서드를 호출합니다.
목록에서 Flyable 및 Car 클래스의 순서를 뒤집으면 그에 따라 __mro__ 가 변경됩니다. 예를 들어:
# Car, Flyable classes...
class FlyingCar(Car, Flyable):
def start(self):
super().start()
if __name__ == '__main__':
car = FlyingCar()
car.start()
print(FlyingCar.__mro__)
Output:
Start the Car
(<class '__main__.FlyingCar'>, <class '__main__.Car'>, <class '__main__.Flyable'>, <class 'object'>)
이 예제에서 super().start()는 MRO에 따라 Car 클래스의 start() 메서드를 대신 호출 합니다.
Multiple inheritance & super
먼저 Car 클래스에 __init__ 메서드를 추가합니다.
class Car:
def __init__(self, door, wheel):
self.door = door
self.wheel = wheel
def start(self):
print('Start the Car')
def go(self):
print('Going')
둘째, __init__ 메서드를 Flyable 클래스에 추가합니다.
class Flyable:
def __init__(self, wing):
self.wing = wing
def start(self):
print('Start the Flyable object')
def fly(self):
print('Flying')
Car 및 Flyable 클래스의 __init__ 다른 수의 매개 변수를 허용합니다. FlyingCar 클래스가 Car 및 Flyable 클래스에서 상속되는 경우 __init__ 메서드는 FlyingCar 클래스의 __mro__에 지정된 올바른 __init__ 메서드를 호출해야 합니다.
셋째, __init__ 메서드를 FlyingCar 클래스에 추가합니다.
class FlyingCar(Flyable, Car):
def __init__(self, door, wheel, wing):
super().__init__(wing=wing)
self.door = door
self.wheel = wheel
def start(self):
super().start()
FlyingCar 클래스의 MRO은 다음과 같습니다.
(<class '__main__.FlyingCar'>, <class '__main__.Flyable'>, <class '__main__.Car'>, <class 'object'>)
super().__init__()는 Flyable 클래스의 __init__ 을 호출합니다 . 따라서 wing 인수를 __init__ 메서드에 전달해야 합니다.
FlyingCar 클래스는 Car 클래스의 __init__ 메서드에 액세스할 수 없으므로 door 및 wheel 속성을 개별적으로 초기화해야 합니다.
Summary
- Multiple inheritance(다중 상속)을 사용하면 한 클래스가 여러 클래스에서 상속할 수 있습니다.
- MRO(메서드 확인 순서)은 호출할 메서드를 찾기 위한 클래스 검색 경로를 정의합니다.
'Python Object-oriented Programming' 카테고리의 다른 글
Data vs. Non-data Descriptors (0) | 2023.04.04 |
---|---|
descriptors (0) | 2023.04.04 |
Interface Segregation Principle (0) | 2023.04.04 |
Liskov Substitution Principle (0) | 2023.04.04 |
Open-closed Principle (0) | 2023.04.04 |
댓글